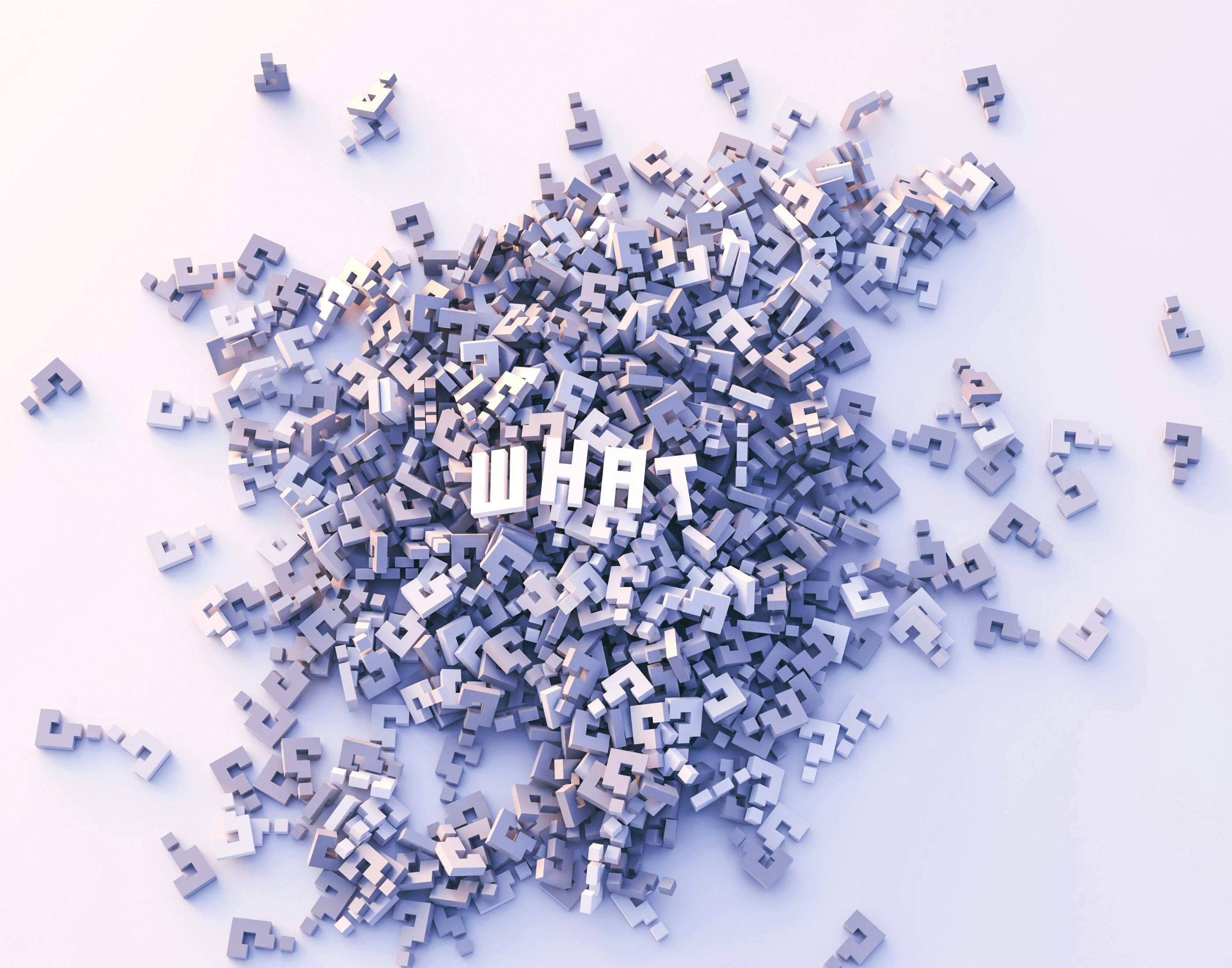
Robotics Job Interview Warm‑Up: 30 Real Coding & System‑Design Questions
Robotics is revolutionising industries—from manufacturing and healthcare to autonomous vehicles and service robots. With the rise of artificial intelligence, computer vision, and mechatronics, modern robots are becoming more capable, adaptive, and intelligent than ever before. If you’re seeking a robotics job, you’ll face a series of interviews that test your knowledge of embedded systems, control theory, software engineering, hardware integration, and more.
In this article, we’ll examine 30 real coding & system-design questions often posed in robotics interviews. We’ll also discuss why targeted interview preparation is crucial for demonstrating both your technical and collaborative skills. And if you’re looking for new robotics roles in the UK, visit www.roboticsjobs.co.uk—a specialised resource for discovering cutting‑edge robotics vacancies, from start-ups developing warehouse automation solutions to large enterprises building autonomous delivery drones.
Let’s start by exploring the unique demands of robotics interviews, and how you can showcase your ability to integrate hardware, software, and advanced algorithms to create robots that operate reliably in the real world.
1. Why Robotics Interview Preparation Matters
Robotics sits at the intersection of mechanical design, electrical engineering, and computer science. Employers expect candidates to blend analytical thinking, coding skills, and hardware experience. Below are some key reasons why interview preparation is essential:
Demonstrate Technical Range
Robotics projects typically involve sensors, actuators, motor control, embedded software, and possibly machine learning or vision.
Interviews probe your competency in multiple domains, from classical control theory to software integration.
Highlight Real‑World Problem‑Solving
Robots operate in dynamic environments, confronting noise, uncertainty, and changing conditions.
Employers want candidates who can tackle practical challenges like sensor fusion, trajectory planning, and robust motion control.
Showcase Collaboration & Communication
Robotics teams often include mechanical engineers, electrical engineers, software developers, data scientists, and UX designers.
You’ll likely be asked about working cross‑functionally, implementing feedback from test operators or other stakeholders.
Emphasise Safety & Reliability
Robots interact with humans and physical objects—safety is paramount.
Interviews may explore your approach to fail‑safes, fault detection, and regulatory compliance (especially for medical or industrial robotics).
Convey Adaptability
Robotics is evolving fast with new sensors, AI models, and embedded platforms hitting the market.
Show that you can learn quickly, evaluate new tools, and pivot design decisions as needed.
Whether you’re building advanced drones or service bots for factories, thorough preparation will help you communicate how your skills, experiences, and problem-solving approaches make you the right fit. Let’s dive into 15 coding questions you might encounter in a robotics interview.
2. 15 Real Coding Interview Questions
Coding Question 1: Motor Control via PWM
Question: Write code (in C/C++ or Python) to output a PWM (Pulse Width Modulation) signal that controls a DC motor’s speed on an embedded platform.
What to focus on:
Initialising timers, configuring PWM frequency and duty cycle,
Adjusting duty cycle in real time to change motor speed,
Possibly using direct hardware registers if on a microcontroller (e.g., Arduino).
Coding Question 2: Sensor Fusion
Question: Implement a function that fuses data from an IMU (Inertial Measurement Unit)—accelerometer and gyroscope readings—to estimate orientation.
What to focus on:
Kalman filter or complementary filter approach,
Handling noise, drift from gyros, dynamic or static motion states,
Converting raw sensor data to Euler angles or quaternions.
Coding Question 3: Kinematic Transformations
Question: Write a function that calculates forward kinematics for a 2‑link planar robotic arm, returning (x, y) of the end effector given joint angles θ1\theta_1θ1 and θ2\theta_2θ2.
What to focus on:
Matrix multiplication or trigonometric expansions,
Summation of angles, link lengths,
Possibly returning orientation if needed.
Coding Question 4: Obstacle Avoidance
Question: Given an array of laser scan readings around a robot, demonstrate how you’d find the minimum distance to any obstacle and steer away if it’s below a threshold.
What to focus on:
Parsing scan data (angles, distances),
Checking thresholds, computing safe direction,
Possibly controlling a differential drive’s linear/angular velocities.
Coding Question 5: ROS Publisher/Subscriber
Question: Show how you’d implement a ROS node that publishes sensor data at 10Hz and subscribes to a command topic to adjust motor speeds.
What to focus on:
Writing a simple
roscpp
orrospy
script,Setting up publishers, subscribers, callback handling,
Rate control (ros::Rate or rospy.Rate) for loop frequency.
Coding Question 6: Path Planning in a Grid
Question: Implement a basic A search* that finds a path from a start cell to a goal cell in a 2D occupancy grid. Return the sequence of grid coordinates.
What to focus on:
Data structures for open/closed sets,
Heuristic function (Manhattan or Euclidean distance),
Reconstructing the path once the goal is found.
Coding Question 7: PID Controller Implementation
Question: Implement a PID controller function that takes in a target setpoint, current value, and returns a control output.
What to focus on:
Summing Proportional, Integral, Derivative terms,
Handling integral windup,
Tuning parameters (Kp, Ki, Kd).
Coding Question 8: Image Processing for Object Detection
Question: Write a snippet that processes a camera image (OpenCV/Python) to detect a coloured marker and return its centroid.
What to focus on:
Converting to HSV colour space, thresholding,
Using
cv2.findContours()
or morphological operations,Calculating the centroid (moments) of the largest blob.
Coding Question 9: Inverse Kinematics
Question: For a 2‑link planar arm, write code that, given (x, y) of the target, computes joint angles θ1\theta_1θ1 and θ2\theta_2θ2.
What to focus on:
Trigonometric approach to solve for angles,
Handling unreachable or multiple solutions,
Checking domain for arccos\arccosarccos, arcsin\arcsinarcsin, or usage of the “law of cosines”.
Coding Question 10: Odometry for a Differential Drive
Question: Implement a function to estimate the robot’s (x, y, θ\thetaθ) using wheel encoder ticks from left and right wheels.
What to focus on:
Translating encoder counts to distance for each wheel,
Calculating rotational changes around the midpoint,
Updating pose in a continuous loop.
Coding Question 11: Task Scheduling on Microcontroller
Question: Demonstrate a simple round-robin scheduler for tasks like sensor reading, motor updates, and communication on a microcontroller (C code).
What to focus on:
Maintaining a timer or tick,
Dividing tasks into time slots or cycling them,
Minimising overhead and ensuring priority tasks run frequently enough.
Coding Question 12: Data Logging & Debug
Question: Write code that logs sensor data to an SD card every second, using minimal overhead, and ensures no data loss if partial writes fail.
What to focus on:
Buffered writes, error checks,
File system approach on embedded system,
Possibly using ring buffers or task scheduling for concurrency.
Coding Question 13: Finite State Machine (FSM) for Robot Behaviours
Question: Implement an FSM in code for a delivery robot that cycles between states: IDLE, NAVIGATE, PICKUP, DELIVER, ERROR.
What to focus on:
Clear transitions, triggers, event handling,
Handling unexpected errors that force transitions to ERROR or IDLE,
State representation (switch statements or function pointers, etc.).
Coding Question 14: Simple SLAM
Question: Outline a high‑level code structure for a 2D EKF (Extended Kalman Filter) SLAM with landmark observations.
What to focus on:
Robot state (x, y, θ\thetaθ) plus landmark positions in the state vector,
Predict step (odometry update),
Update step (range/bearing from landmarks),
Covariance matrix management.
Coding Question 15: Multi‑Threaded Control
Question: Demonstrate how you’d structure a multi‑threaded application that runs a real‑time control loop, a sensor reading loop, and a high‑level planner.
What to focus on:
Using concurrency libraries (C++ std::thread, Python threading, or real-time OS tasks),
Thread synchronisation, priority, or using message queues,
Minimising latency for control tasks.
These coding questions cover fundamental robotics software tasks: sensor integration, motion control, planning, concurrency, and more. Next, let’s explore the system design side of robotics, which often involves multi-layer architectures spanning hardware, firmware, and high-level software.
3. 15 System & Architecture Design Questions
Design Question 1: Robot Operating System (ROS) Architecture
Scenario: You’re building a mobile robot with multiple sensors (LIDAR, camera, IMU). Outline a ROS‑based system architecture that integrates all nodes, transforms, and a navigation stack.
Key Points to Discuss:
Separate nodes for sensor drivers, navigation, and motion control,
tf tree for coordinate transforms,
Using
move_base
or custom nav stack with costmaps, local/global planners.
Design Question 2: Autonomous Warehouse Robot
Scenario: A robot must navigate aisles, pick items, and transport them. How do you design a system that handles path planning, obstacle avoidance, and picking tasks?
Key Points to Discuss:
Multi‑layer architecture: perception (map building), planning (route scheduling), manipulation (arm for picking),
Scheduling tasks in a queue, integration with warehouse management system,
Safety sensors, fallback if aisle is blocked.
Design Question 3: Sensor Fusion Architecture
Scenario: You have LIDAR, depth camera, and wheel odometry. Propose a system that fuses these to produce a stable pose estimate for an indoor robot.
Key Points to Discuss:
Extended Kalman Filter or particle filter for multi‑sensor data,
Handling sensor timing, out‑of‑sync frames,
Weighted approach if one sensor is unreliable (e.g., camera in low light).
Design Question 4: Remote Teleoperation
Scenario: You want to remotely operate a robot in a hazardous environment. Discuss communication channels, latency handling, and operator UI design.
Key Points to Discuss:
Network constraints (bandwidth, potential disconnects),
Streaming video vs. sensor data,
Operator input device (joystick, VR), safety override or e‑stop.
Design Question 5: Robot Arm on a Mobile Base
Scenario: A manipulator is mounted on a mobile robot. Outline how you’d unify the coordinate systems and ensure stable operation when the base moves.
Key Points to Discuss:
Transform frames between base, arm joints, end‑effector,
Possible sensor feedback to detect base motion,
Integration of mobile manipulation: scheduling arm motion with stable base posture.
Design Question 6: Safety and Redundancy
Scenario: A collaborative robot in a factory must safely pause if a human enters its workspace. Design the system and logic.
Key Points to Discuss:
Proximity sensors, light curtains, or vision to detect humans,
Immediate motor torque reduction or e‑stop signal,
Resuming tasks once area is clear, logging events for safety compliance.
Design Question 7: Vision‑Based SLAM Platform
Scenario: You have a stereo camera to perform visual SLAM (e.g., ORB‑SLAM). How do you structure the pipeline to ensure real‑time performance on embedded hardware?
Key Points to Discuss:
Feature extraction, matching, local map updates,
Loop closure detection,
Possibly offloading heavy tasks to GPU or using a hardware accelerator.
Design Question 8: High‑Level Robot Fleet Management
Scenario: Multiple robots operate in a large facility. Propose a system to coordinate tasks, avoid collisions, and track their status.
Key Points to Discuss:
Central cloud or local server scheduling tasks (jobs, routes),
Robot–robot communication or a global manager that assigns paths,
Handling dynamic changes, conflict resolution if two robots want the same corridor.
Design Question 9: Real‑Time OS vs. Linux
Scenario: You’re designing a control system for a medical robot. Should you use a real‑time OS or a standard Linux OS with a real‑time patch?
Key Points to Discuss:
Latency and determinism requirements,
Tools and ecosystem for building real‑time scheduling,
Certification or regulatory aspects for safety.
Design Question 10: Autonomous Car Architecture
Scenario: Design a software stack for a self‑driving car: from sensor input (LiDAR, radar, cameras) to motion planning and actuation.
Key Points to Discuss:
Perception pipeline (object detection/tracking),
Behaviour planning (lane changes, merging),
Low‑level control (steering, throttle, braking), failover strategies.
Design Question 11: Edge vs. Cloud for Robot Intelligence
Scenario: A service robot with limited CPU can offload heavy computations (SLAM, deep learning) to the cloud. Discuss pros and cons.
Key Points to Discuss:
Latency, potential network outages,
Data privacy or bandwidth constraints,
Hybrid solution: partial edge inference, partial cloud for heavy tasks.
Design Question 12: Robotics Simulation & Testing
Scenario: Propose a pipeline for developing and validating a new robot in simulation (Gazebo or Webots) before physical tests.
Key Points to Discuss:
Building URDF or SDF models,
Simulating sensors, testing control algorithms,
Transition strategy for real hardware, calibration differences between sim and reality.
Design Question 13: Manipulation Task Planning
Scenario: A robot arm must pick items of various shapes from a bin. Discuss how you’d handle object detection, grasp planning, and motion execution.
Key Points to Discuss:
3D vision or point cloud processing for object segmentation,
Grasp detection (suction or parallel grippers, possibly ML-based grasping),
MoveIt or custom inverse kinematics for collision-free path planning.
Design Question 14: Low‑Power Mobile Robot Design
Scenario: A battery-powered robot must run for 8 hours autonomously. Outline how you’d manage power consumption across motors, sensors, and onboard computer.
Key Points to Discuss:
Sleep modes for idle sensors,
Efficient motor drivers (BLDC with advanced control),
Intelligent scheduling so CPU-intensive tasks don’t run continuously.
Design Question 15: Data Logging & Telemetry
Scenario: During field tests, you need to log sensor data (IMU, GPS, camera) plus system states. Propose an architecture for storing, syncing, and retrieving logs.
Key Points to Discuss:
Local storage vs. real-time streaming to a remote server,
Time-synchronised data across multiple sources,
Tools for post-processing (ROS bag files, custom DB solutions).
These system design scenarios test your ability to engineer integrated robotics solutions across sensors, actuators, software frameworks, and safety/regulatory constraints. Now let’s cap things off with practical interview tips for robotics roles.
4. Tips for Conquering Robotics Job Interviews
Brush Up on Core Robotics Concepts
Revisit topics like kinematics, dynamics, control theory (PID, LQR), path planning (RRT, A)*, and sensor fusion (Kalman filters).
If you’re AI-focused, confirm your knowledge of computer vision, machine learning, or reinforcement learning for robotics.
Show Code & Project Portfolios
If you’ve built personal projects (e.g., a line‑following robot), highlight them.
Demos or GitHub repos can illustrate real-world experience.
Discuss Safety & Reliability
Real robots can injure people or damage property if they fail.
Emphasise your approach to safety: E‑stops, redundancy, design checks, software testing, especially in collaborative or medical environments.
Highlight Cross‑Functional Experience
Robotics merges hardware, software, electronics, and mechanical design.
Show how you communicate with teams of different engineering backgrounds.
Remember Realistic Constraints
Robots face battery limits, weight constraints, sensor noise, calibration drift.
If asked about system design, talk about trade‑offs (precision vs. cost, heavier battery vs. longer runtime).
Stay Current with Tools & Frameworks
ROS, Gazebo, MoveIt are common for robotics software. Also consider MATLAB/Simulink, OpenCV, or microcontroller toolchains.
Reinforcement learning might use PyTorch or TensorFlow.
Prepare to Answer “How Did You Debug?”
Robotics frequently breaks in surprising ways—be ready to discuss your debugging steps, data logging, or real‑time monitoring approaches.
Ask Informed Questions
Inquire about the company’s product roadmap, test environment, hardware choices, or collaboration with external partners.
Shows genuine interest and helps you gauge project scale, team structure, or engineering culture.
Master Communication & Demos
If there’s a technical presentation, practise explaining complex robots or algorithms in simple terms.
Visual aids or short videos of your prototypes can make a strong impression.
Embrace Continuous Learning
Robotics is fast‑moving. Show you’re open to new sensors, new computing platforms (NVIDIA Jetson, RISC‑V boards), or AI methods.
Demonstrate adaptability—companies value those who can pivot quickly.
By blending robust technical foundations, hands‑on project experience, and a team-oriented mindset, you’ll position yourself as a top candidate in the robotics field.
5. Final Thoughts
Robotics offers a challenging, exciting career—bringing together hardware, software, AI, and design to solve real-world problems. By reviewing the 30 questions in this article—spanning coding tasks and system‑level architecture—you’ll sharpen the skills that top employers seek: from PID control and path planning to multi‑sensor fusion and complex system integration.
Couple your preparation with real-world projects, a solid understanding of safety standards, and a readiness to collaborate across disciplines—and you’ll be well on your way to acing your next robotics interview. For the latest robotics opportunities in the UK, explore www.roboticsjobs.co.uk. There, you’ll find roles in diverse fields, including warehouse automation, healthcare robotics, autonomous vehicles, and more.
With thorough study, a problem-solving mindset, and clear communication, you’ll stand out as a future robotics innovator—building the machines that reshape how we live and work.